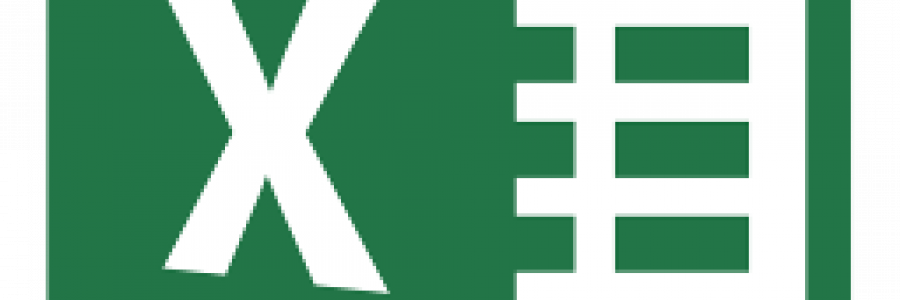
MS-Excel Stream Handler is a PHP Class, This class implements a stream handler interface to read and write Microsoft Excel spreadsheet files in the XLS format.
XLS files may be read and written using PHP fopen, fread or fwrite, or any other PHP functions that can access to streams. The file name has the format xlsfile://path/to/spreadsheet.xls where /path/to/spreadsheet.xls is the path of the actual spreadsheet file to be read or written.
To create a XLS file just pass a serialized array of spreadsheet row arrays that associate the column names with their values.
In the current version the class does not process the data read from a XLS file. Instead it just returns the raw XLS file data as is.
This class requires at least PHP 4.3.0 as that is the version when stream handling support was introduced in PHP.
You can create a MS-Excel file in the following way,
//Include downloaded the excel php file.
require_once "excel.php";
$fp = fopen("xlsfile://tmp/test.xls", "wb");
if (!is_resource($fp)) {
die("Cannot open excel file");
}
$data= array(
array("Name" => "Bob Loblaw", "Age" => 50),
array("Name" => "Popo Jijo", "Age" => 75),
array("Name" => "Tiny Tim", "Age" => 90)
);
fwrite($fp, serialize($data));
fclose($fp);
And the following code will read the excel files,
header ("Content-Type: application/x-msexcel");
header ("Content-Disposition: attachment; filename=\"sample.xls\"" );
readfile("xlsfile://path/to/sample.xls");
exit;